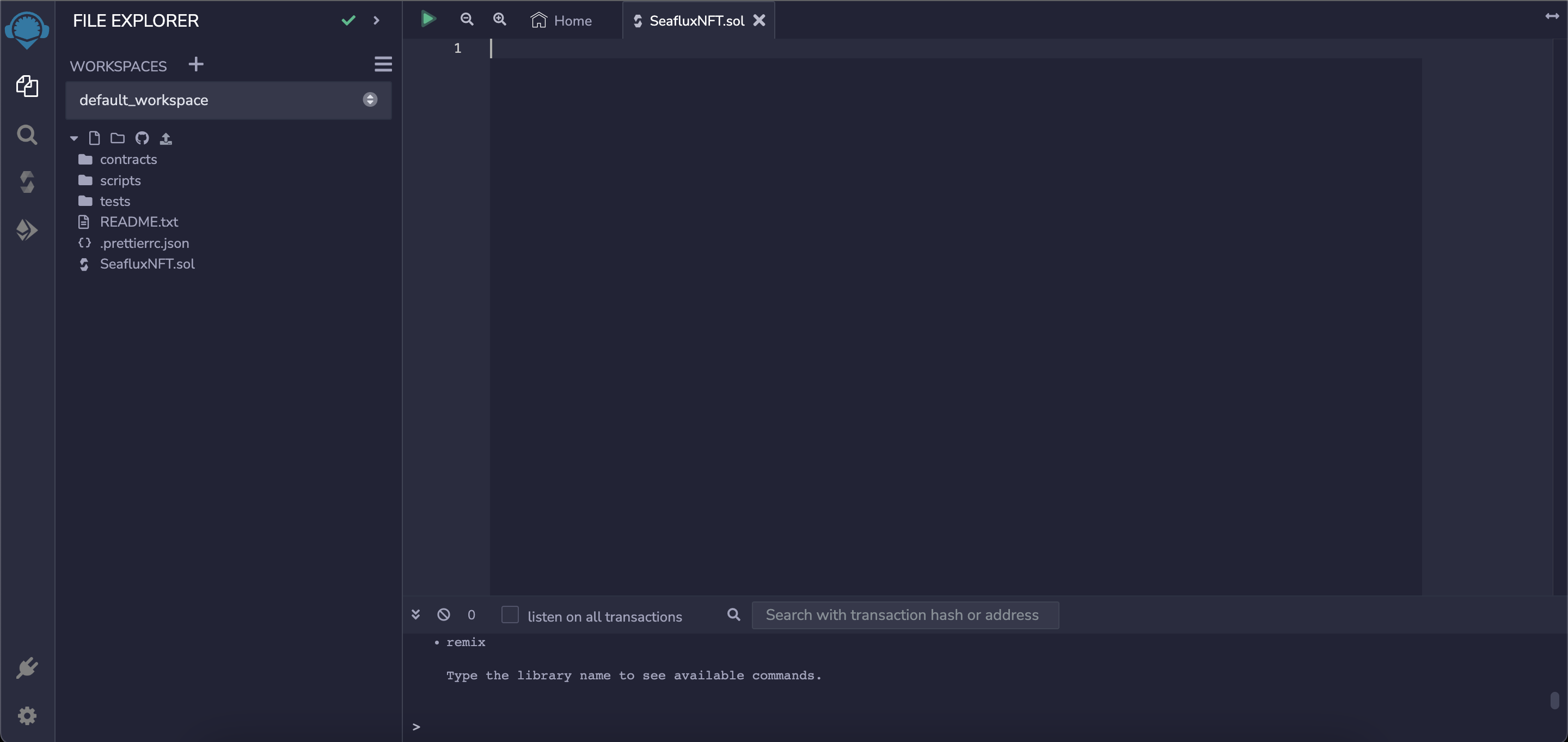
Non-Fungible means the things that hold some uniqueness, and are not interchangeable with similar items. Similarly, NFT (Non-Fungible Tokens) are the tokens used to define ownership of unique items. These items can be art, videos, audio, 3D models, or even real estate.
We have already shown you how to create tokens using ERC20 smart contract. We also have a particular blog based on Flow Blockchain, where you can learn how to Mint NFT on Flow Blockchain. In this blog, we will see how to develop an ERC1155 NFT smart contract and mint our very first NFT. We are going to use Remix IDE for the development and testing of the contract.
Firstly, open Remix and create a new file named SeafluxNFT.sol as shown in the image below.
Now, we will add the SPDX License Identifier and the Solidity version (We are using 0.8.11 for this contract). Next, we import a few modules provided by OpenZeppelin and add the declaration for the contract. Modules are required to import the methods of a contract standard, say ERC1155 or ERC20, or else, we need to write the abstract methods on our own.
// SPDX-License-Identifier: MIT
pragma solidity 0.8.11;
import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/token/ERC1155/extensions/ERC1155Burnable.sol";
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
contract SeafluxNFT is ERC1155, Ownable, ERC1155Burnable {
}
A few pre-requisite are required before we proceed with the smart contract.
We have imported the following libraries in the above code:
We have declared a contract named SeafluxNFT which inherits the ERC1155, ERC1155Burnable, and Ownable modules.
Next, we are going to declare the following variables:
contract SeafluxNFT is ERC1155, Ownable, ERC1155Burnable {
uint256 public mintPrice = 0.001 ether;
string public name = "Seaflux NFT";
uint256 public maxSupply=10000;
uint256 internal totalMinted;
}
Now let's add a constructor in our code to initialize the ERC1155 class by passing in the IPFS path of our NFT, which will be used to display our NFT once it is minted. A constructor is a method commonly used in Object Oriented Programming and gets called whenever a class is initialized. In Solidity contracts, however, the constructor is called only once: when the contract is deployed.
constructor()
ERC1155("https://ipfs.io/ipfs/QmRyFNM4yS2Le6FHMb8KoFq7276NBNPZd5Hc5VmnukUoWT")
{}
Next, we will add the following functions to the contract:
function mint(uint256 numOfNFTs) external payable {
require(totalMinted + numOfNFTs undefined maxSupply,"Minting would exceed max supply");
require(mintPrice * numOfNFTs undefined= msg.value,"Not enough MATIC sent");
require(numOfNFTs undefined= 10,"Only up to 10 NFTs can be minted");
_mint(msg.sender, 1, numOfNFTs, "");
totalMinted += numOfNFTs;
}
function getTotalSupply() external view returns (uint256) {
return maxSupply;
}
function getMinted() external view returns (uint256) {
return totalMinted;
}
function withdraw() public onlyOwner {
require(address(this).balance undefined 0);
uint256 contractBalance = address(this).balance;
payable(_msgSender()).transfer(contractBalance);
}
After adding all the functions, the contract will look like this:
// SPDX-License-Identifier: MIT
pragma solidity 0.8.11;
import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/token/ERC1155/extensions/ERC1155Burnable.sol";
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
contract SeafluxNFT is ERC1155, Ownable, ERC1155Burnable {
uint256 public mintPrice = 0.001 ether;
string public name = "Seaflux NFT";
uint256 public maxSupply=10000;
uint256 internal totalMinted;
constructor()
ERC1155("https://ipfs.io/ipfs/QmRyFNM4yS2Le6FHMb8KoFq7276NBNPZd5Hc5VmnukUoWT")
{}
function mint(uint256 numOfNFTs) external payable {
require(totalMinted + numOfNFTs undefined maxSupply,"Minting would exceed max supply");
require(mintPrice * numOfNFTs undefined= msg.value,"Not enough MATIC sent");
require(numOfNFTs undefined= 10,"Only up to 10 NFTs can be minted");
_mint(msg.sender, 1, numOfNFTs, "");
totalMinted += numOfNFTs;
}
function getTotalSupply() external view returns (uint256) {
return maxSupply;
}
function getMinted() external view returns (uint256) {
return totalMinted;
}
function withdraw() public onlyOwner {
require(address(this).balance undefined 0);
uint256 contractBalance = address(this).balance;
payable(_msgSender()).transfer(contractBalance);
}
}
Now, head over to the Compile section in the Remix and check for any issues in the contract.
Once the contract is successfully compiled, move it to the Deploy section. Select the Inject Provider - Metamask
Next, click on the Deploy button, which will deploy the contract on the selected testnet/mainnet.
Once the contract is deployed, we will mint 1 NFT. Add 1 as the argument for the mint function. This NFT is priced at 0.001 ETH, which is equal to 1 Finney. Make sure to send in 1 Finney with the transaction. Now call the mint function and wait for the transaction to go through.
Once the transaction goes through, head on to the Testnet Opensea and login with your Metamask. Select the address with which you minted the NFT and open the profile page. You will see your first-ever minted NFT in the Collected tab.
We have now minted our first NFT in your Metamask wallet. We used Remix to write all the Solidity code. We saw the variables that were used while deploying the smart contract and what are its functions, along with writing the codes for the contract. Now kindly head over to Remix and mint your first NFT.
We, at Seaflux, are Blockchain enthusiasts who are helping enterprises worldwide. Have a query or want to discuss Blockchain projects? Schedule a meeting with us here, we'll be happy to talk to you!
Director of Engineering