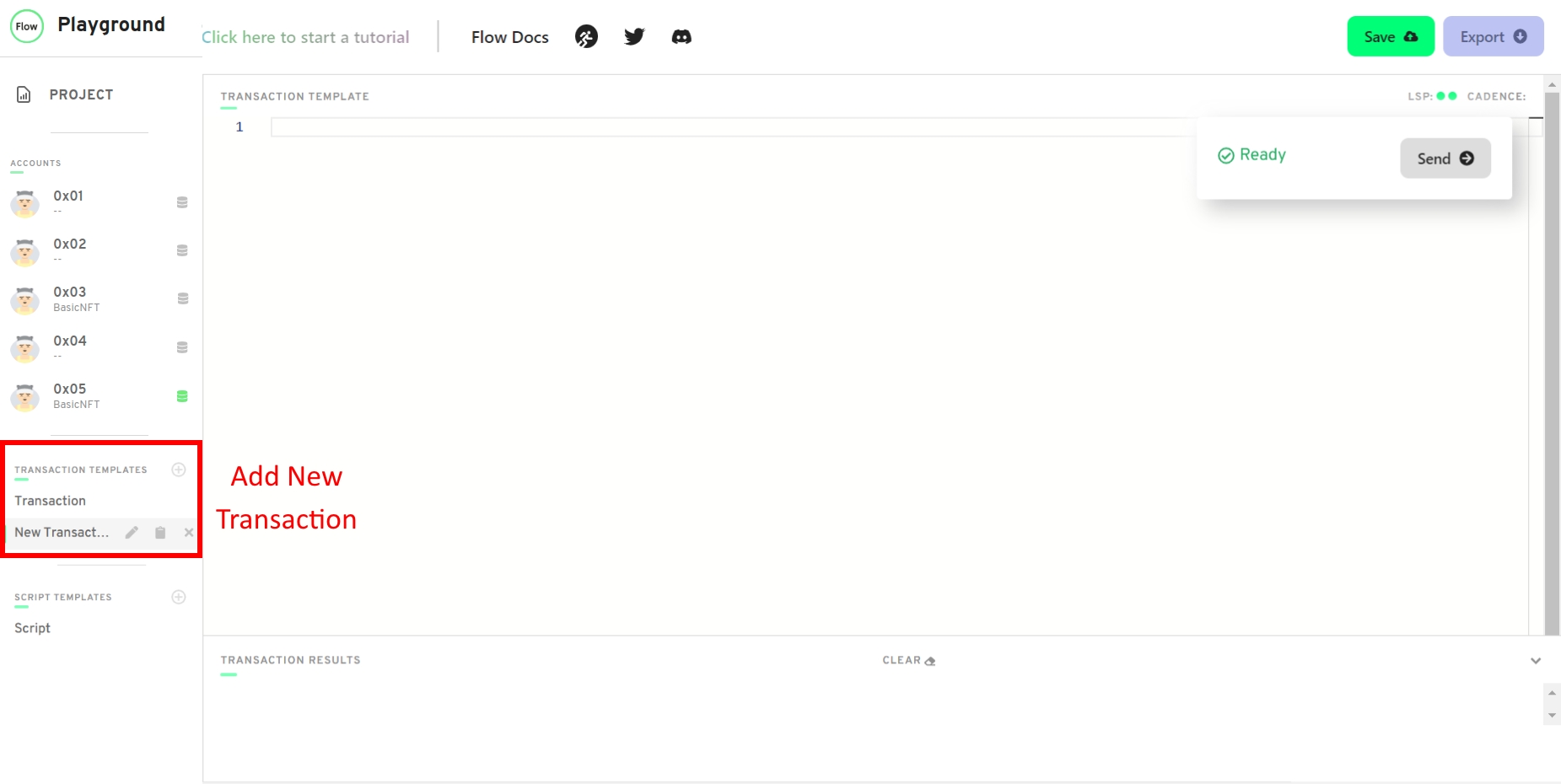
Flow blockchain has become one of the most preferred choices when it comes to developing an entertainment related NFT or project. The ecosystem works at a low transaction cost and a user-centric experience, which is luring more users and developers to work with the blockchain. We have covered the basics of the Flow Blockchain in our previous blog, kindly head over to that blog if you haven't already.
In this blog, we will see:
Prerequisite:
Tools:
First, open the flow playground, where the smart contracts for Flow are being written, to write your NFT smart contract.
Here, we create BasicNFT smart contract, which has an init() function, which is called every time when the smart contract is deployed.
pub contract BasicNFT{
pub var minted: UInt64
init(){
self.minted = 0
}
}
Now, create an NFT resource. Resources are types that can only exist in one location at a time and must be used exactly once. So at the end of the function, in which resources are used, we need to move or destroy the resource.
pub contract BasicNFT{
pub var minted: UInt64
init(){
self.minted = 0
}
pub resource NFT{
pub let id: UInt64
pub var metadata: {String: String}
init(InitURL: String){
self.id = BasicNFT.minted
self.metadata = {"URL": InitURL}
BasicNFT.minted = BasicNFT.minted + 1
}
}
}
Here, in the resource, we have two variables: id and metadata. As the name suggests, the id variable will store the unique ID for a particular NFT, and the metadata variable will hold the metadata URL for that NFT.
In the init() function, when a resource is called, it increases the total supply value by 1. So every time, we get a unique ID for the NFT.
We also create two functions, nft_url and nft_id, to get the URL and ID for the NFT minted on the flow blockchain.
pub fun getID(): UInt64{
return self.id
}
pub fun getURL(): String{
return self.metadata["URL"]!
}
Now, we create a createNFT() function that takes metadata as input to create an NFT resource.
pub fun createNFT(url: String): @NFT{
return undefined- create NFT(InitURL: url)
}
Here, we create a resource interface NFTPublic to call the getID and getURL functions from the NFT resource and give this NFTPublic type to resource NFT.
pub resource interface NFTPublic{
pub fun getID(): UInt64
pub fun getURL(): String
}
Now, our smart contract is ready for deployment. After doing all the steps your smart contract looks like this.
pub contract BasicNFT{
pub var minted: UInt64
init(){
self.minted = 0
}
pub resource interface NFTPublic{
pub fun getID(): UInt64
pub fun getURL(): String
}
pub resource NFT: NFTPublic{
pub let id: UInt64
pub var metadata: {String: String}
init(InitURL: String){
self.id = BasicNFT.minted
self.metadata = {"URL": InitURL}
BasicNFT.minted = BasicNFT.minted + 1
}
pub fun getID(): UInt64{
return self.id
}
pub fun getURL(): String{
return self.metadata["URL"]!
}
}
pub fun createNFT(url: String): @NFT{
return undefined- create NFT(InitURL: url)
}
}
Now, after creating a smart contract, we deploy it on the account provided by flow playground. After deploying the smart contract, we create a transaction script to call the createNFT() function from the smart contract and mint NFT on our account.
In the playground compiler, there is an option called 'transaction'. Click on add icon and create a new script as createNFT(). In this script, import your smart contract from the account in which you deployed it.
Now we will add a transaction that takes the metadata URL as input.
import BasicNFT from 0x05
transaction (url: String){
prepare(acct: AuthAccount) {
acct.save(undefined-BasicNFT.createNFT(url: url), to: /storage/BasicNFTPath)
acct.linkundefinedundefinedBasicNFT.NFT{BasicNFT.NFTPublic}undefined(/public/BasicNFTPath, target: /storage/BasicNFTPath)
}
execute {
log("NFT Created!")
}
}
Here, the transaction script calls the creatNFT() function of the smart contract, and when NFT is minted, it saves the NFT in storage. Also, link the smart contract NFTPublic interface with the account used for minting the NFT.
After running the script NFT is created.
And it's stored in your wallet. You can get the NFT id and NFT URL by creating a new script that calls the smart contract function getID() and getURL().
Below is the script to get the NFT details.
import BasicNFT from 0x05
pub fun main(account: Address): AnyStruct {
let publicReference = getAccount(account).getCapability(/public/BasicNFTPath) .borrowundefinedundefinedBasicNFT.NFT{BasicNFT.NFTPublic}undefined()
?? panic("No NFT reference found here!")
return [publicReference.getID(), publicReference.getURL()]
}
Now by running the script you get your NFT details. The output of the following code is as shown below:
To summarize, we covered below steps:
We, at Seaflux, are Blockchain enthusiasts who are helping enterprises worldwide. Have a query or want to discuss Blockchain projects? Schedule a meeting with us here, we'll be happy to talk to you.
Director of Engineering