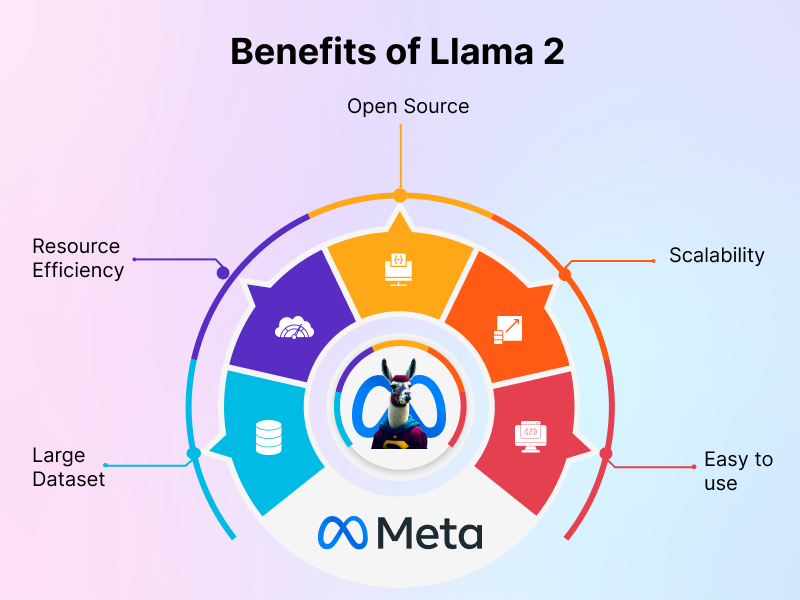
Llama 2 is an open-source large language model (LLM) developed by Meta and Microsoft. Llama 2 stands for large language model by Meta AI. If you want to understand a large language model, you can visit another blog called What is LLM? Understanding with Examples. Llama 2 is based on the Transformer architecture, which is the same architecture used by other popular LLMs such as GPT-3.
Meta AI Llama 2 is trained on a massive dataset of text and code. This dataset includes text from books, articles, code repositories, and other sources. The size of the dataset varies depending on the version of Llama 2. The smallest version, Llama 2 7B Chat, is trained on a dataset of 7 billion words. The largest version, Llama 2 70B Chat, is trained on a dataset of 70 billion words.
The different versions of Llama 2 are distinguished by the size of the dataset they are trained on as well as the specific tasks they are designed for. The larger the dataset, the more powerful the model will be. The specific tasks that a model is designed for will also affect its performance. For example, a model that is designed for question answering will be better at answering questions than a model that is designed for text generation.
Ultimately, the best version of Llama 2 for a particular task will depend on the specific requirements of that task. If you are not sure which version to use, you can consult the Meta website for more information.
To incorporate Llama 2 into your project, it's essential to acquire access to the Llama 2 model from the Hugging Face library. The following steps outline how to obtain access to Llama 2 using the Hugging Face platform.
Once you get access to Llama 2, you can use the below code for implementation.
While you have the option to write the code in any IDE or Google Colab, it's advisable to use Google Colab for coding, as it provides a distinct advantage due to its provision of a free GPU.
In case you've come across our earlier blog on LLM, referenced in the introduction section, it's worth noting that we utilize the transformer library for model training. To proceed with this, you'll need to install the transformer library and some other required packages.
!pip install transformers
!pip install huggingface_hub
!pip install accelerate
!pip install xformers
Following the installation of this library, the next step involves logging into Hugging Face using a token that you must generate on the Hugging Face website.
from huggingface_hub import notebook_login
notebook_login()
Once you execute the command mentioned earlier, a prompt will surface. Inside this prompt, you have to enter your access token. After the token is successfully verified, you will be able to integrate the Llama 2 model into your code.
Here, we are utilizing the Llama-2-7b-chat-hf model in this context, which allows you to operate within the confines of Colab's free tier, provided that you opt for a GPU runtime.
Firstly, you need to import all the required packages that are used to train the model.
from transformers import AutoTokenizer
import transformers
import torch
Now, you need to write the model name in the below command.
model = "meta-llama/Llama-2-7b-chat-hf"
In the below code, we are loading a pre-trained tokenizer from the Hugging Face model hub and passing our Llama model in it.
tokenizer = AutoTokenizer.from_pretrained(model)
Now let’s start with the process of building our text-generation pipeline, which involves the integration of the Llama 2 model. This configuration, along with the specified torch_dtype (16-bit floating-point precision) and device_map (automatic device selection), will enable the pipeline to undertake text-generation tasks effectively. The torch_dtype setting influences the data type used for computations, optimizing memory usage and speed, while the device_map parameter ensures that the computations are executed on the appropriate device (CPU or GPU) without manual intervention.
pipeline = transformers.pipeline(
"text-generation",
model=model,
torch_dtype=torch.float16,
device_map="auto",
)
We can add our text to the above-created pipeline by using the below code, with the do_sample parameter set to True, the pipeline generates text while considering multiple possibilities. The top_k parameter limits the vocabulary choices to the top 10 most likely tokens. Only one generated sequence is returned due to num_return_sequences=1. The eos_token_id specifies the end-of-sequence token from the tokenizer, ensuring the generated text doesn't exceed 210 tokens as defined by max_length.
sequences = pipeline(
Having enjoyed novels like 'To Kill a Mockingbird' and '1984', could you suggest any other books that align with my preferences?',
do_sample=True,
top_k=10,
num_return_sequences=1,
eos_token_id=tokenizer.eos_token_id,
max_length=210,
)
for seq in sequences:
print(f"Result: {seq['generated_text']}")
Result: Having enjoyed novels like 'To Kill a Mockingbird' and '1984', could you suggest any other books that align with my preferences? 🤔"
The user has a preference for novels that have a strong narrative, engaging characters, and explore themes of social justice, morality, and the human condition. They have enjoyed novels that tackle difficult issues and are looking for more books that share similar characteristics.
Books that might be of interest to this user include:
1. 'The Catcher in the Rye' by J.D. Salinger: This classic novel explores themes of alienation, disillusionment, and the struggle to find one's place in the world.
2. 'The Handmaid's Tale' by Margaret Atwood: Set in a dystopian future, this novel explores a society where women have lost their rights and are forced into reproductive servitude.
These are just a few of the tasks that the different versions of Llama 2 can be used for. As Llama 2 continues to develop, it is likely that it will be able to do even more things.
In conclusion, Llama 2 is a powerful open-source language model by Meta AI, based on the Transformer architecture. It offers various versions tailored for specific tasks, while its accessibility and efficiency benefit researchers and developers. Challenges include resource demands, bias, and safety concerns. Llama 2 applications range from text generation to chatbots, holding promise for further advancements in language processing.
We, at Seaflux, are AI undefined Machine Learning enthusiasts, who are helping enterprises worldwide. Have a query or want to discuss AI projects where Llama 2 can be leveraged? Schedule a meeting with us here, we'll be happy to talk to you.
Director of Engineering