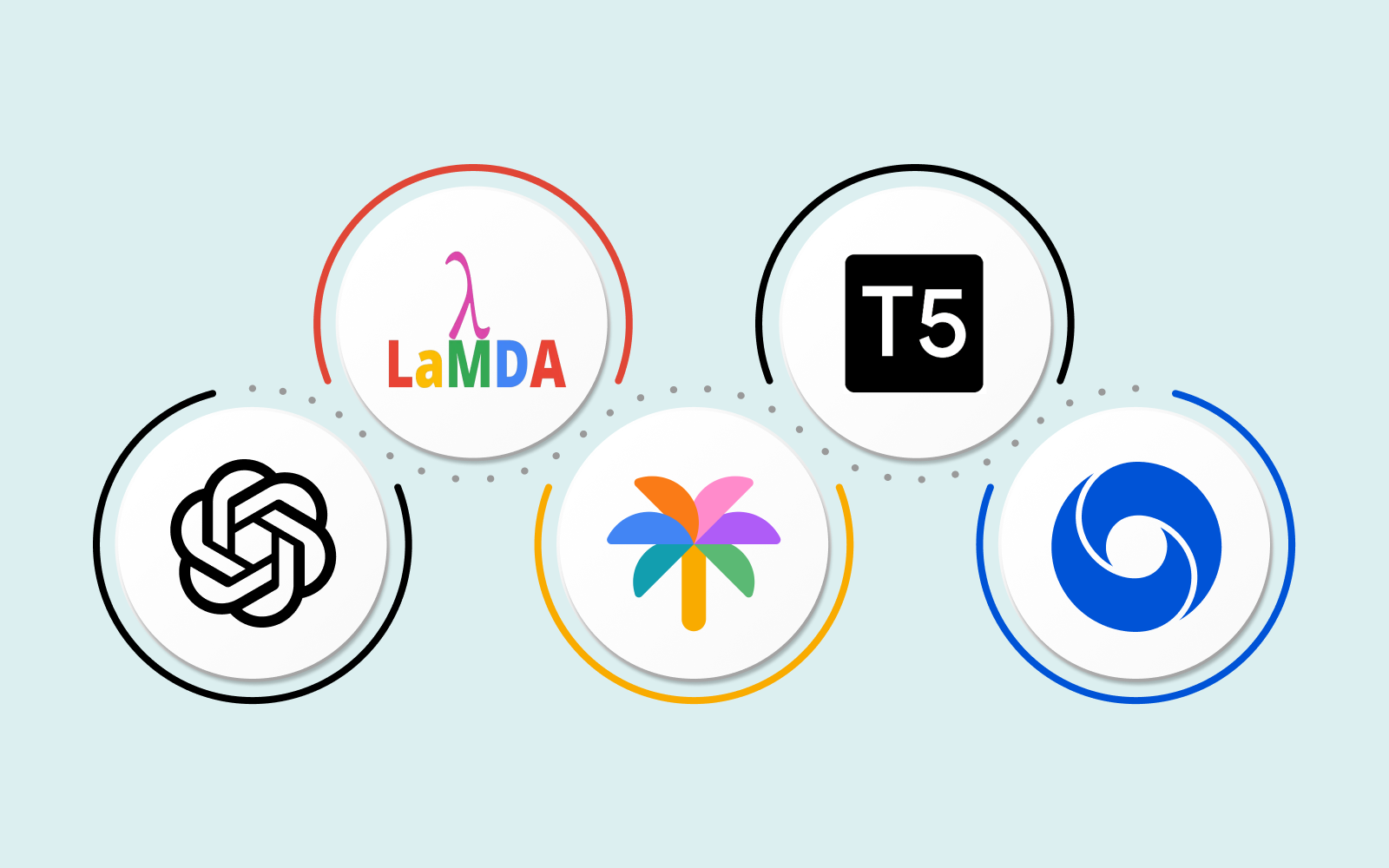
Since ancient times, language has been important to learning and communication. It has constantly evolved to see what it is now, with a variety found all over the world. With the advancement in technology, it's not surprising to see AI understanding and answering human conversations with such precision.
This is possible with the Large Language Model being implemented in the latest openAI's ChatGPT and Google's Bard systems. The machine learning branch is an emerging technology, and has a long way to go and ease our lives. Let us go through what LLMs are, some examples, its advantages, challenges, and use cases.
LLM (Large Language Model) is a type of AI model designed to understand and generate human-like text. These models are trained on vast amounts of text data and use deep learning techniques, such as deep neural networks, to process and generate language.
LLMs are capable of performing various natural language processing (NLP) tasks, including
They are trained on a wide range of textual data sources, such as books, articles, websites, and other written content, allowing them to learn grammar, vocabulary, and contextual relationships in language.
Some of the most popular large language models are:
Now that we have gone through the examples of Large Language Models, let us see how to utilize an LLM Library in different use cases along with code build. The LLM library used is provided by Hugging Face, called Transformer Library.
The transformer package, provided by huggingface.io, tries to solve the various challenges we face in the NLP field. It provides pre-trained models, tokenizers, configs, various APIs, ready-made pipelines for our inference, etc.
It is a large language model (LLM) developed by Hugging Face and a community of over 1000 researchers. It is trained on a massive dataset of text and code, and it can generate text, translate languages, and answer questions. Here we are going to see the following application of the Transformer Library:
Sentiment Analysis | Named Entity Recognition |
Text Generation | Translate language |
Question Answering Pipeline | Summarization |
Before jumping to the examples of Transformer Library, we need to install the library to use it.
pip install transformers
By using the pipeline feature of the Transformers Library, you can easily apply LLMs for text generation, question answering, sentiment analysis, named entity recognition, translation, and more.
from transformers import pipeline
To perform sentiment analysis using the Transformers library, you can utilize the pipeline feature with a pre-trained sentiment analysis model. Here's an example:
from transformers import pipeline
classifier = pipeline("sentiment-analysis")
res = classifier("I have been waiting for a hugging face course my whole life.")
print(res)
We get the following output from the code:
Output - [{'label': 'POSITIVE', 'score': 0.9980935454368591}]
In this example, we used the pipeline function with the "sentiment-analysis" task to load a pre-trained sentiment analysis model. The sentiment_analyzer pipeline takes the input text and outputs the sentiment label and score, indicating the sentiment polarity (positive, negative, or neutral) and the confidence level of the sentiment prediction.
Let’s look at some more examples using other LLMs.
To complete the sentence using Large Language Models. For getting the best response we are describing large language models using the gpt2 model. Here's an example:
from transformers import pipeline
generator = pipeline("text-generation", model="distilgpt2")
res = generator(
"In this course, we will teach you to",
max_length=30,
num_return_sequences=2,
)
print(res)
We get the following output from the code:
Output - [{'generated_text': 'In this course, we will teach you to make sure you understand the importance of a number of aspects of technology, including the way in which we know'}, {'generated_text': 'In this course, we will teach you to create and manage all our applications and manage our work on a shared platform. This course will teach you your'}]
In this example, we used the pipeline function with the "text-generation" task to load a pre-trained text-generation model. The text_generator pipeline takes the prompt as input and generates text based on the model's understanding of the language. The max_length parameter limits the length of the generated text, and num_return_sequences controls the number of generated sequences to return.
To perform question-answering using the Transformers library, you can utilize the pipeline feature with a pre-trained question-answering model. Here's an example:
from transformers import pipeline
# Define the list of file paths
file_paths = ['document1.txt', 'document2.txt', 'document3.txt']
# Read the contents of each file and store them in a list
documents = []
for file_path in file_paths:
with open(file_path, 'r') as file:
document = file.read()
documents.append(document)
# Concatenate the documents using a newline character
context = "\n".join(documents)
# Use the pipeline with the updated context
nlp = pipeline("question-answering")
result = nlp(question="When did Mars Mission Launched?", context=context)
print(result['answer'])
The code prints the below output correctly to the question – When did Mars Mission Launch?
Output - 5 November 2013
In this example, the code defines a list of file paths that can be any document of any format like Txt, pdf, URLs, multiple documents in string formats, and so on. It then reads the contents of each file and stores them in a list. These documents are concatenated using a newline character.
The next step is to create a pipeline for question-answering. The pipeline is initialized with the "question-answering" task. The pipeline can then be used to answer questions about the context. In this example, the question is "When did Mars Mission Launched?" The answer to the question is printed on the console.
We can summarize using Large Language Models. Let’s summarize a long text describing large language models using the t5 model. Here's an example:
from transformers import pipeline
context = r """ The Mars Orbiter Mission (MOM), also called Mangalyaan ("Mars-craft", from Mangala, "Mars" and yāna, "craft, vehicle") is a space probe orbiting Mars since 24 September 2014? It was launched on 5 November 2013 by the Indian Space Research Organisation (ISRO). It is India's first interplanetary mission and it made India the fourth country to achieve Mars orbit, after Roscosmos, NASA, and the European Space Company. and it made India the first country to achieve this in the first attempt. The Mars Orbiter took off from the First Launch Pad at Satish Dhawan Space Centre (Sriharikota Range SHAR), Andhra Pradesh, using a Polar Satellite Launch Vehicle (PSLV) rocket C25 at 09:08 UTC on 5 November 2013. The launch window was approximately 20 days long and started on 28 October 2013. The MOM probe spent about 36 days in Earth orbit, where it made a series of seven apogee-raising orbital before trans-Mars injection on 30 November 2013 (UTC).[23] After a 298-day long journey to Mars orbit, it was put into Mars orbit on 24 September 2014."""
summarizer = pipeline(
"summarization", model="t5-base", tokenizer="t5-base", framework="tf")
summary = summarizer(context, max_length=130, min_length=60)
print(summary)
The output will print the summarized text about LLMs:
[{'summary_text': "The Mars Orbiter Mission (MOM) is a space probe orbiting Mars since 24 September 2014. It is India's first interplanetary mission and it made India the fourth country to achieve Mars orbit. the probe spent about 36 days in Earth orbit before trans-Mars injection on 30 November 2013 ."}]
To translate text using the Transformers library, you can utilize the pipeline feature with a pre-trained translation model. Here's an example:
from transformers import pipeline
en_fr_translator = pipeline("translation_en_to_fr")
text = "How old are you?"
translation = en_fr_translator(text, max_length=500)
result = translation[0]["translation_text"]
print(result)
The output will print “How old are you?” in the French language
Output - quel âge êtes-vous?
In this example, we used the pipeline function with the "translation" task to load a pre-trained translation model. The translator pipeline takes the input text and generates the translation based on the specified model. The max_length parameter limits the length of the translated text.
To perform named entity recognition (NER) using the Transformers library, you can utilize the pipeline feature with a pre-trained NER model. Here's an example:
from transformers import pipeline
# Load the pre-trained NER model
ner = pipeline("ner")
# Define the text for named entity recognition
text = "Apple Inc. was founded by Steve Jobs, Steve Wozniak, and Ronald Wayne."
# Perform named entity recognition using the transformer model
entities = ner(text)
# Extract the named entities and their corresponding labels from the model's response
for entity in entities:
entity_text = entity["word"]
entity_label = entity["entity"]
print(f"Entity: {entity_text}, Label: {entity_label}")
The output will print the list of entities with their label
Output - Entity: Apple Inc., Label: ORG
Entity: Steve Jobs, Label: PERSON
Entity: Steve Wozniak, Label: PERSON
Entity: Ronald Wayne, Label: PERSON
In this example, the model has identified the named entities "Apple Inc." as an organization (ORG), and "Steve Jobs," "Steve Wozniak," and "Ronald Wayne" as persons (PERSON).
Large language models (LLMs) have a number of advantages over traditional machine learning models. These advantages include:
Large language models (LLMs) are a powerful new technology, but they also come with several challenges. These challenges include:
The future of LLM models is bright. As this technology continues to develop, we can expect to see even more innovative and groundbreaking applications for LLMs in the future.
Some of the promising applications of LLMs include:
Now we have understood what Large Language Models are and how you can leverage the Transformer Library with six examples. We have seen the advantages, challenges, and use cases. Do you plan to use the LLM with your business as well? Seaflux has is well equipped in the arena to make it happen. Contact us and let us work for you to make your life simple.
We, at Seaflux, are AI undefined Machine Learning enthusiasts, who are helping enterprises worldwide. Have a query or want to discuss AI projects where Mojo and Python can be leveraged? Schedule a meeting with us here, we'll be happy to talk to you.
Director of Engineering