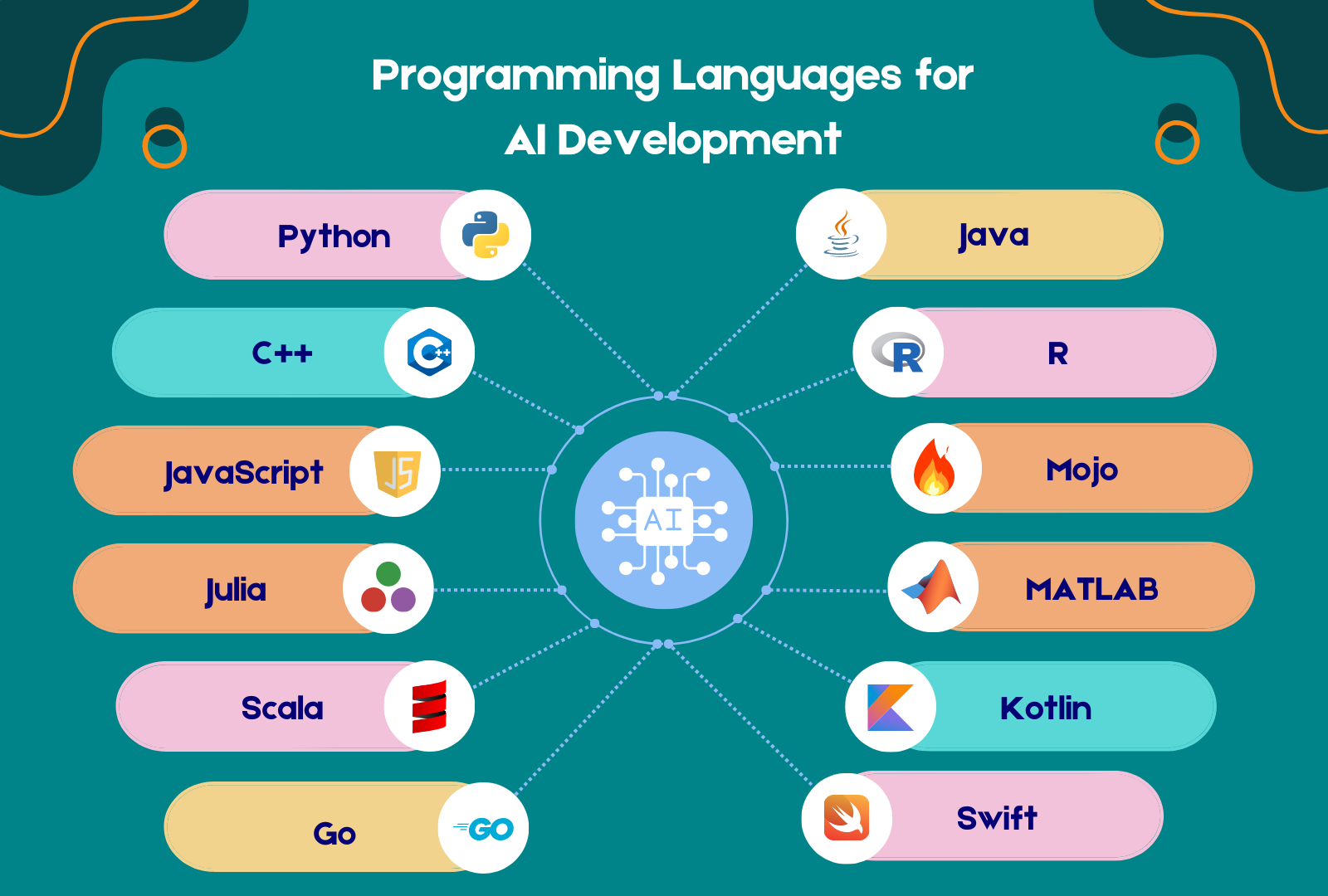
Artificial Intelligence (AI) has become a dominant force in various industries, revolutionizing the way we live and work. From virtual assistants to self-driving cars, AI-powered technologies have permeated every aspect of our lives. If you're interested in exploring the world of AI development, it's crucial to understand the essential programming languages that form the foundation of this field. In this article, we will delve into the top programming languages for AI development and explore their unique features and applications.
This article covers a range of programming languages used in AI development. The following table provides a list of programming languages commonly used in AI development:
Each language offers unique features and strengths for different aspects of AI, providing developers with a variety of options to suit their needs and preferences. These programming languages play a vital role in AI development, empowering developers to create intelligent systems and applications.
Python stands as the undisputed heavyweight champion when it comes to programming languages for AI development. Its simplicity, versatility, and vast ecosystem of libraries make it the go-to choice for AI enthusiasts and professionals alike. Python's readability and clean syntax make it an excellent language for both beginners and experienced developers.
Python enables you to utilize libraries like TensorFlow, Keras, and PyTorch for constructing and training intricate neural networks. These libraries offer a user-friendly interface and robust tools for deep learning, a vital component of AI development. Furthermore, Python's extensive support for scientific computing and data analysis makes it a preferred option for machine learning assignments.
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
Java, a widely adopted programming language, is renowned for its scalability, performance, and extensive ecosystem. While Java may not be the primary choice for AI development, it plays a significant role in developing AI applications that require high performance and large-scale processing.
Java's multithreading and concurrent programming capabilities empower developers to leverage parallel computing, a crucial element of AI algorithms. Moreover, Java's reliable machine learning libraries, such as Deeplearning4j (DL4J), offer resources for constructing deep learning models using Java's object-oriented approach.
import org.deeplearning4j.datasets.iterator.impl.MnistDataSetIterator;
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork;
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deeplearning4j.nn.conf.layers.DenseLayer;
import org.nd4j.linalg.activations.Activation;
import org.nd4j.linalg.learning.config.Adam;
import org.nd4j.linalg.lossfunctions.LossFunctions;
MnistDataSetIterator mnistTrain = new MnistDataSetIterator(batchSize, true, rngSeed);
NeuralNetConfiguration.Builder builder = new NeuralNetConfiguration.Builder();
builder.updater(new Adam())
.activation(Activation.RELU)
.l2(1e-4)
.list()
.layer(new DenseLayer.Builder().nIn(784).nOut(256).build())
.layer(new DenseLayer.Builder().nIn(256).nOut(64).build())
.layer(new OutputLayer.Builder(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD)
.activation(Activation.SOFTMAX)
.nIn(64).nOut(outputNum).build());
MultiLayerNetwork model = new MultiLayerNetwork(builder.build());
model.init();
model.fit(mnistTrain);
C++ has long been known for its efficiency and control over system resources, making it a popular choice for performance-critical applications. In the realm of AI development, C++ finds its place in building highly optimized AI algorithms and frameworks.
C++'s utilization of low-level features, including pointers and manual memory management, empowers developers to optimize their AI implementations for optimal performance. Furthermore, C++ offers a vast array of libraries, such as OpenCV and Eigen, which provide robust tools for computer vision and linear algebra – critical components in AI development.
#include undefinediostreamundefined
#include undefinedopencv2/opencv.hppundefined
int main() {
cv::Mat image = cv::imread("image.jpg");
cv::cvtColor(image, image, cv::COLOR_BGR2GRAY);
cv::GaussianBlur(image, image, cv::Size(5, 5), 0);
cv::Canny(image, image, 50, 150);
cv::imshow("Edge Detection", image);
cv::waitKey(0);
return 0;
}
JavaScript, the language of the web, has been expanding its reach into the AI landscape with the advent of libraries and frameworks like TensorFlow.js and Brain.js. With JavaScript, developers can now build AI applications that run directly in web browsers.
JavaScript's widespread usage and seamless integration with HTML and CSS make it a perfect selection for creating AI-driven web applications, interactive visualizations, and chatbots. By leveraging pre-trained models and APIs, JavaScript empowers developers to harness the capabilities of AI without requiring in-depth understanding of the underlying algorithms.
const model = tf.sequential();
model.add(tf.layers.dense({ units: 64, activation: 'relu', inputShape: [784] }));
model.add(tf.layers.dense({ units: 10, activation: 'softmax' }));
model.compile({ optimizer: 'adam', loss: 'categoricalCrossentropy', metrics: ['accuracy'] });
While Python takes the limelight in the AI world, R shines brightly in the realm of statistics and data analysis. R boasts a comprehensive collection of statistical libraries and packages, making it a preferred choice for researchers and statisticians working in AI development.
R's emphasis on statistical modeling and visualization tools, exemplified by the ggplot2 library, enables data scientists to extract valuable insights from intricate datasets. Moreover, R's strong integration with databases and other programming languages, such as Python, makes it a superb option for data manipulation and preprocessing operations.
library(ggplot2)
data undefined- read.csv("data.csv")
ggplot(data, aes(x=age, y=salary, color=gender)) + geom_point()
Mojo is a new programming language that is designed to be scalable and high-performance for AI applications. It combines the usability of Python with the performance of C, unlocking unparalleled programmability of AI hardware and extensibility of AI models.
Mojo is built on top of MLIR, a modern compiler infrastructure that enables Mojo to target a wide range of hardware platforms, including CPUs, GPUs, and TPUs. This makes Mojo ideal for developing AI applications that need to scale to large datasets and models.
Mojo also has a rich set of features that make it well-suited for AI development, including:
Mojo is a promising new programming language for AI development. It is scalable, high-performance, and has a rich set of features that make it well-suited for AI applications.
import mojo.nn as nn
import mojo.dataset as ds
# Load the MNIST dataset
mnist = ds.MNIST()
# Create a neural network
model = nn.Sequential(
nn.Dense(784, 256),
nn.ReLU(),
nn.Dense(256, 10),
nn.Softmax()
)
# Train the model
model.fit(mnist, epochs=10)
For more details about Mojo and to explore the power of this programming language, you can refer to the blog post Exploring the Power of Mojo🔥 Programming Language. This blog delves deeper into Mojo's scalability, high-performance capabilities, and its rich set of features tailored for AI development.
Julia is a relatively new programming language that has gained attention in the AI community for its high-performance capabilities. Julia offers a combination of dynamic programming and high-level abstractions, making it an attractive choice for AI researchers and developers.
Julia excels in its seamless integration with pre-existing code written in languages like C, Python, and R. The just-in-time (JIT) compilation feature of Julia ensures efficient execution and impressive performance, rendering it well-suited for computationally demanding AI tasks.
using Flux
model = Chain(
Dense(784, 256, relu),
Dense(256, 64, relu),
Dense(64, 10),
softmax
)
loss(x, y) = Flux.crossentropy(model(x), y)
MATLAB, often associated with scientific and engineering applications, also finds its place in AI development. MATLAB's rich set of built-in functions, toolboxes, and visualization capabilities make it an invaluable asset for AI researchers and practitioners.
MATLAB offers a user-friendly interface and comprehensive documentation, which facilitates a seamless learning curve for individuals venturing into the realm of AI. With its robust matrix manipulation capabilities, MATLAB simplifies intricate mathematical operations inherent in AI algorithms. Furthermore, MATLAB's Deep Learning Toolbox provides indispensable functions for constructing and training deep learning models.
data = readtable('data.csv');
X = data(:, 1:3);
y = data(:, 4);
model = fitlm(X, y);
Scala, a language that combines object-oriented and functional programming paradigms, offers a unique perspective on AI development. Scala's interoperability with Java allows developers to leverage existing Java libraries and frameworks, making it a versatile choice for AI applications.
Scala's inclusion of functional programming features, such as immutable data structures and higher-order functions, establishes a sturdy groundwork for constructing AI algorithms. Its concise and expressive syntax empowers developers to write code that is both clean and elegant, all while ensuring scalability and performance.
import org.apache.spark.ml.classification.LogisticRegression
import org.apache.spark.ml.feature.VectorAssembler
import org.apache.spark.sql.SparkSession
val spark = SparkSession.builder()
.appName("AI App")
.getOrCreate()
val data = spark.read.format("csv")
.option("header", "true")
.load("data.csv")
val assembler = new VectorAssembler()
.setInputCols(Array("feature1", "feature2", "feature3"))
.setOutputCol("features")
val transformedData = assembler.transform(data)
val lr = new LogisticRegression()
.setLabelCol("label")
.setFeaturesCol("features")
val model = lr.fit(transformedData)
Kotlin, a modern programming language that runs on the Java Virtual Machine (JVM), offers a blend of simplicity and expressiveness. With its concise syntax and null safety features, Kotlin provides a more intuitive and safer programming experience for AI developers.
Kotlin's compatibility with Java facilitates effortless integration with established Java libraries and frameworks, thereby broadening the horizons for AI development. With its functional programming capabilities, including higher-order functions and lambda expressions, Kotlin simplifies the implementation of AI algorithms and facilitates the management of intricate data transformations.
import org.jetbrains.kotlinx.dl.api.core.Sequential
import org.jetbrains.kotlinx.dl.api.core.layer.Dense
import org.jetbrains.kotlinx.dl.api.core.layer.Input
import org.jetbrains.kotlinx.dl.api.core.layer.ReLU
import org.jetbrains.kotlinx.dl.api.core.layer.Softmax
import org.jetbrains.kotlinx.dl.api.core.layer.convolutional.Conv2D
import org.jetbrains.kotlinx.dl.api.core.optimizer.Adam
val model = Sequential.of(
Input(784),
Dense(256, activation = ReLU()),
Dense(64, activation = ReLU()),
Dense(10, activation = Softmax())
)
model.compile(optimizer = Adam(), loss = CrossEntropy())
model.fit(xTrain, yTrain, batchSize = 64, epochs = 10)
Go, also known as Golang, is a programming language that emphasizes simplicity, efficiency, and ease of use. While not as widely used in AI development as Python or R, Go offers a unique approach to building AI applications.
Go's simplicity and clear syntax make it an excellent option for creating AI algorithms that demand efficient execution and high performance. Its built-in concurrency primitives, such as goroutines and channels, enable parallel processing, rendering it well-suited for AI tasks involving extensive datasets and intricate computations.
import (
"fmt"
"github.com/sjwhitworth/golearn/base"
"github.com/sjwhitworth/golearn/ensemble"
)
instances, err := base.ParseCSVToInstances("data.csv", true)
if err != nil {
fmt.Println(err)
}
classifier, err := ensemble.NewRandomForest(10, 4)
if err != nil {
fmt.Println(err)
}
classifier.Fit(instances)
Swift, developed by Apple, has gained popularity as the go-to language for iOS and macOS app development. While primarily associated with mobile and desktop applications, Swift has also found its place in AI development within the Apple ecosystem.
Developers can utilize Swift to unlock the potential of Apple's machine learning framework, Core ML, seamlessly integrating AI functionalities into their applications. Swift's contemporary syntax, type safety, and comprehensive standard library make it a fitting option for AI tasks encompassing data preprocessing, model deployment, and inference on Apple devices.
import CreateMLUI
let builder = MLImageClassifierBuilder()
builder.showInLiveView()
As the field of AI continues to advance at a rapid pace, the choice of programming language becomes crucial for developers and researchers. Python stands tall as the powerhouse of AI, offering a versatile and extensive ecosystem of libraries. R excels in statistical modeling and data analysis, while Java, Julia, MATLAB, Scala, Kotlin, Go, Swift, C++, JavaScript, and Mojo each bring their unique strengths and applications to AI development.
By understanding the essential programming languages for AI development, you can equip yourself with the right tools and knowledge to embark on this exciting journey. Whether you prioritize simplicity, performance, scalability, or integration, there's a programming language that suits your needs in the vast landscape of AI development.
We, at Seaflux, are AI undefined Machine Learning enthusiasts, who are helping enterprises worldwide. Have a query or want to discuss AI projects where these languages can be leveraged? Schedule a meeting with us here, we'll be happy to talk to you.
Director of Engineering